Elixir Programming
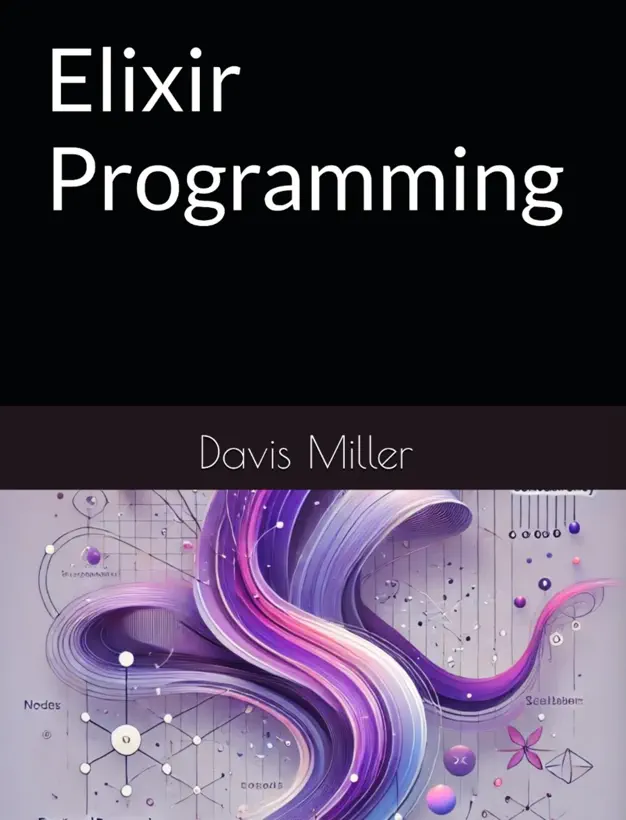
Buy the Book
You can buy the complete book with detailed explanations, code examples on:
Amazon Paperback
Kindle Edition
Amazon Hardcover
Google Play Books
Audiobook
Apple Book
Books to Read
Welcome to Elixir Programming, a comprehensive guide designed to help you master Elixir, a dynamic, functional language known for its scalability and fault-tolerant capabilities. Whether you’re a seasoned developer exploring functional programming or a newcomer looking for a modern language to build scalable systems, this book offers a structured approach to understanding and leveraging Elixir’s powerful features.
Elixir stands out in the programming landscape for its elegant syntax, seamless concurrency model, and its foundation on the robust Erlang VM (BEAM). These qualities make it a top choice for building applications that demand high availability, such as real-time systems, distributed applications, and microservices.
Table of Contents
Introduction to Elixir
- What is Elixir?
- The Erlang VM (BEAM)
- Why Elixir? Benefits and Use Cases
- Conclusion
Setting Up the Elixir Environment
- Installing Elixir
- Basic Tools: iex, mix, and Hex
- Writing Your First Elixir Program
- Conclusion
Core Concepts
- Data Types and Pattern Matching
- Functions and Modules
- Control Structures and Flow
- Conclusion
Core Functional Concepts
- Immutability
- Higher-Order Functions
- Closures and Anonymous Functions
- Conclusion
Enumerables and Streams
- Working with Lists and Maps
- Enum and Stream Modules
- Lazy Evaluation and Pipelines
- Conclusion
Recursion and Tail-Call Optimization
- Understanding Recursion
- Writing Tail-Recursive Functions
- Best Practices
- Conclusion
The Actor Model
- Understanding the Actor Model
- Processes in Elixir
- Conclusion
Tasks and Agents
- Asynchronous Programming with Tasks
- Using Agents for Shared State
- Best Practices
- Conclusion
OTP Framework
- Introduction to OTP (Open Telecom Platform)
- GenServer: Building Stateful Processes
- GenStage and Flow for Data Processing
- Conclusion
Distributed Systems
- Running Elixir on Multiple Nodes
- Clustering and Distributed Computing
- Conclusion
Fault Tolerance and Resilience
- Handling Failures Gracefully
- Monitoring and Debugging
- Best Practices
- Conclusion
Web Development with Phoenix
- Introduction to Phoenix Framework
- Building REST APIs and Real-Time Applications
- Phoenix LiveView for Interactive UIs
- Best Practices
- Conclusion
Working with Databases
- Introduction to Ecto
- Setting Up Ecto
- Using Ecto for Database Access
- Queries
- Changesets
- Migrations
- Example: Building a User Management System
- Best Practices
- Conclusion
- Introduction to Metaprogramming
- Understanding Macros
- Writing Your Own Macros
- Example: Building a Domain-Specific Language (DSL) with Macros
- Testing Macros
- Best Practices
- Conclusion
Testing in Elixir
- Introduction to Testing in Elixir
- Unit Testing with ExUnit
- Property-Based Testing
- Conclusion
- Introduction to Performance Optimization
- Profiling and Benchmarking
- Tuning Elixir Applications
- Example: Optimizing a GenServer
- Conclusion
Interoperability
- Introduction to Interoperability
- Interfacing with Erlang
- Calling Native Code
- Conclusion
Deployment
- Introduction to Deployment in Elixir
- Packaging and Deploying Elixir Applications
- Using Distillery and Mix Releases
- Containerization with Docker
- Example: Deploying an Elixir Application
- Conclusion
Building a Complete Application
- Introduction
- Designing a Full-Stack Elixir Application
- Implementing the Full-Stack Elixir Application
- Integrating with Third-Party APIs
- Deployment Considerations
- Best Practices
- Conclusion