Learn C++
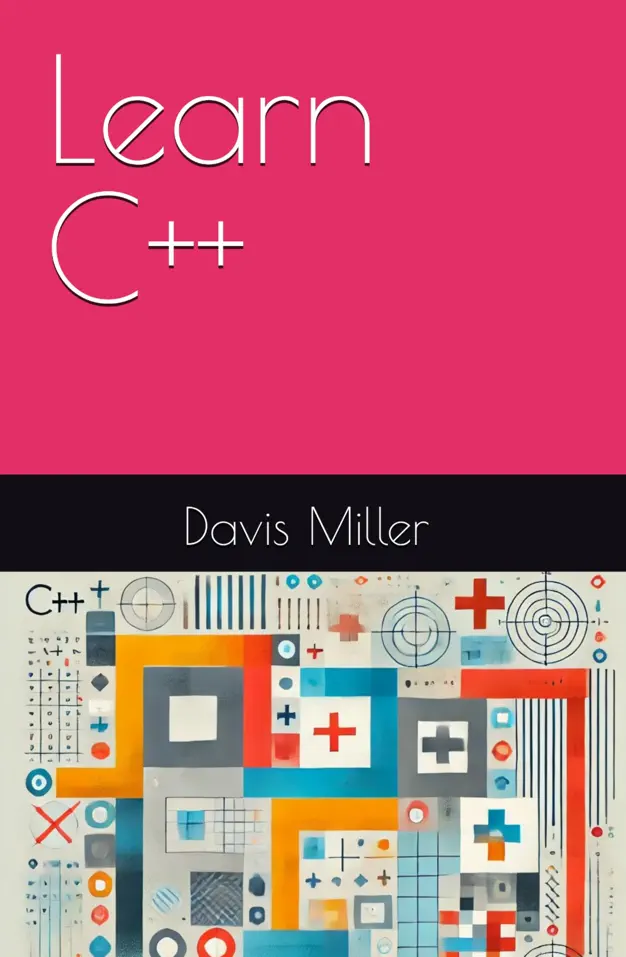
Buy the Book
You can buy the complete book with detailed explanations, code examples on:
Amazon Paperback
Kindle Edition
Google Play Books
Audiobook
Apple Book
Books to Read
C++ remains one of the most influential and widely used programming languages in the software development industry, renowned for its performance, versatility, and powerful feature set. This book is crafted to guide both beginners and experienced programmers through the intricate world of C++, starting with its rich history and evolution from the C language to the modern standards that define it today. You will explore the fundamental concepts of C++, including its object-oriented programming capabilities, efficient memory management, and the extensive Standard Library that empowers developers to build robust and high-performance applications. From setting up your development environment to writing and debugging your first program, each chapter is designed to build a solid foundation in C++ programming.
As you progress, the book delves deeper into advanced topics such as templates, exception handling, and operator overloading, enabling you to write generic and resilient code. Modern C++ features introduced in C++11 and beyond, including smart pointers, concurrency, and compile-time programming, are thoroughly covered to ensure you stay current with the latest industry practices. Additionally, comprehensive sections on the Standard Template Library (STL), memory management strategies, and essential debugging and testing methodologies provide you with the tools necessary to develop sophisticated and maintainable software solutions. Whether your goal is to create high-performance applications, engage in system-level programming, or master the latest C++ techniques, this book serves as your indispensable companion on the journey to mastering C++.
Table of Contents
Introduction to “Learn C++” by Davis Miller
- Introduction to C++
- History and Evolution of C++
- Features and Advantages of C++
- Object-Oriented Programming (OOP)
- Performance and Efficiency
- Rich Standard Library
- Compatibility with C
- Advantages of C++ Features and Compatibility
- Conclusion
Setting up the Development Environment
- Installing Compilers
- Integrated Development Environments (IDEs) Overview
- Configuring Build Systems
Writing and Running Your First C++ Program
- Hello World Example
- Compilation and Execution Process
- Understanding Compiler Errors and Warnings
- Conclusion
Basic Syntax and Structure
- C++ Program Structure
- Source Files and Headers
- The
main
Function
- Comments and Documentation
- Conclusion
Data Types and Variables
- Fundamental Types (
int
, char
, float
, etc.)
- Type Modifiers (
signed
, unsigned
, long
, etc.)
- Constants and Literals
- Variable Declarations and Initialization
- Variable Scope and Lifetime
- Summary and Conclusion
C++ Operators
- Arithmetic, Relational, and Logical Operators
- Bitwise Operators
- Assignment and Compound Operators
- Operator Precedence and Associativity
- Summary
Control Structures
- Conditional Statements
- Loops
- Jump Statements
- Summary
Functions
- Function Declaration and Definition
- Parameter Passing
- Default and Variadic Parameters
- Function Overloading and Inline Functions
- Additional Concepts
- Best Practices
- Summary
Object-Oriented Programming (OOP)
- Classes and Objects
- Defining Classes
- Creating Objects
- Member Variables and Functions
- Summary
Constructors and Destructors
- Types of Constructors
- Destructor: Role and Usage
- Initialization Lists
- Summary
Inheritance
- Base and Derived Classes
- Access Specifiers (
public
, protected
, private
)
- Multiple and Virtual Inheritance
- Comprehensive Example: Inheritance in C++
- Key Concepts and Best Practices
- Summary
Polymorphism
- Understanding Polymorphism in C++
- Compile-Time Polymorphism
- Run-Time Polymorphism
- The
virtual
Keyword and Dynamic Binding
- Summary
Encapsulation and Abstraction
- Encapsulation in C++
- Abstraction in C++
- Hiding Implementation Details
- Summary
Advanced C++ Features
Templates
- Function Templates
- Class Templates
- Template Specialization and Partial Specialization
- Variadic Templates
- Summary
Exception Handling
- Try, Catch, and Throw
- Standard Exception Classes
- Custom Exception Types
- Summary
Operator Overloading
- Overloading Unary and Binary Operators
- Overloading Stream Operators (
<<
, >>
)
- Summary
Namespaces
- Defining and Using Namespaces
- The
std
Namespace
- Nested Namespaces and Aliasing
- Summary
The C++ Standard Library
- Overview of Components
- Importance of the STL in C++ Programming
- Practical Example Combining STL Components
- Summary
Containers
- Sequence Containers
- Associative Containers
- Container Adapters
- Choosing the Right Container
- Practical Examples
- Summary
Iterators
- Iterator Categories
- Iterator Operations
- Iterator Invalidation
- Practical Examples
- Summary
STL Algorithms
- Sorting, Searching, and Modifying Algorithms
- Using Algorithms with Iterators
- Custom Predicates and Function Objects
- Summary
Function Objects and Lambda Expressions
- Defining and Using Function Objects
- Lambda Expressions: Syntax and Usage
- Captures and Closures
- Advanced Topics
- Summary
Utility Components
std::pair
and std::tuple
std::optional
, std::variant
, and std::any
- Smart Pointers (
std::unique_ptr
, std::shared_ptr
, std::weak_ptr
)
- Summary
Modern C++ (C++11 and Beyond)
Auto and Type Inference
- Introduction to Type Inference
- The
auto
Keyword
- The
decltype
Keyword
- The
decltype(auto)
Specifier
- Comparison and When to Use What
Move Semantics and Rvalue References
- Introduction to Move Semantics and Rvalue References
- Understanding Lvalues vs. Rvalues
- Move Constructors and Move Assignment
- Understanding
std::move
and std::forward
- Best Practices and Common Pitfalls
- Comparison: Copy Semantics vs. Move Semantics
- Conclusion
Smart Pointers in C++
- Introduction to Smart Pointers
- Memory Management with Smart Pointers
- Custom Deleters
- Best Practices and Common Pitfalls
- Comparison: Smart Pointers vs. Raw Pointers
- Conclusion
Concurrency and Multithreading
- Threads and Thread Management
- Mutexes, Locks, and Condition Variables
- Atomic Operations and Memory Models
- Best Practices and Considerations
- Conclusion
Memory Management
- Dynamic Memory Allocation
new
and delete
Operators
- Allocators and Custom Memory Management
- Best Practices and Considerations
- Conclusion
Pointers and References in C++
- Raw Pointers vs. Smart Pointers
- Pointer Arithmetic
- References vs. Pointers
- Best Practices and Considerations
- Conclusion
Resource Acquisition Is Initialization (RAII)
- Managing Resources with RAII
- Implementing RAII in Classes
- Benefits for Exception Safety
- Best Practices and Considerations
- Conclusion
Advanced Topics
- Template Metaprogramming Techniques
constexpr
Metaprogramming
- Best Practices and Considerations
- Conclusion
C++17 Features
- Structured Bindings
std::optional
, std::variant
, std::any
- Parallel Algorithms
- Filesystem Library
- Additional C++17 Features
C++20 Features
- Concepts and Ranges
- Modules
- Coroutines
- Enhanced Lambdas
- Additional C++20 Features
- Conclusion
C++23 Features
- Latest Language Enhancements
- Library Additions
- Deprecations and Removals
- Compiler and Tooling Support
- Migration and Compatibility
- Conclusion
Debugging and Testing
Debugging
- Using Debuggers
- Setting Breakpoints and Watchpoints
- Analyzing Stack Traces and Core Dumps
- Best Practices and Techniques for Effective Debugging
- Conclusion
Unit Testing
- Introduction to Testing Frameworks
- Writing and Organizing Test Cases
- Test-Driven Development (TDD) Principles
- Best Practices for Unit Testing in C++
- Conclusion